In Spring ’10 Salesforce released new Apex functionality for aggregate functions. Prior to this feature being available, one would have to perform a large query, loop through it and perform calculations themselves to do things like count records, sum values, get the max value, etc. Now, you can do it in one simple, fast query!
The API Guide has all the details about how to perform aggregate functions in SOQL, but at a high-level, they (along with the GROUP BY clause) let you do things like:
- Get a count of Accounts grouped by Industry & State
- Get the avg Opportunity amount grouped by Calendar Month
- Get the sum of Custom Object $ field grouped by Account
- etc.
Get the idea? The functions you have available are COUNT(), COUNT_DISTINCT(), AVG(), SUM(), MIN(), MAX(). Think of these like you would the Columns To Total in a summary report.
You can also use the GROUP BY clause in your query to summarize your data by other fields. Think of it like a Summary Report grouping.
The best way to learn it is to put it into practice. To do so, I am going to “upgrade” the code from my Campaign Member Summary using Google Charts post. The goal is to create a chart that looks like below:
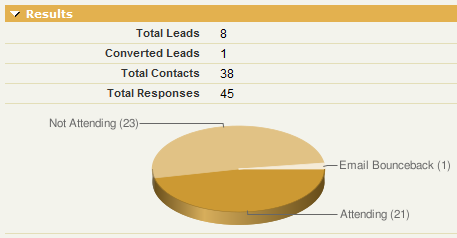
The original controller used the following code to build the count of records per Campaign Member Status:
// List of valid Campaign Member Statuses for the Campaign
List<CampaignMemberStatus> list_cms = [select Id, Label from CampaignMemberStatus where CampaignId = :camp.id];
// Loop through each Campaign Member Status, get a count of Campaign Members and add it to the items list
for (CampaignMemberStatus cms:list_cms) {
integer Count = [select count() from CampaignMember where CampaignId = :camp.id AND Status = :cms.Label];
if (Count > 0) {
items.add(new ChartDataItem(cms.Label, Count.format()));
}
}
The code above works just fine, but has some issues. The main issue is that we are performing a query inside a for() loop. Bad! If a Campaign had more than 20 statuses, the code would fail. Also, this is a performance hit because we have to perform as many queries as there are member statuses. Enter aggregate functions.
The improved controller changes the query to work like this.
// Get all the data in one query
AggregateResult[] groupedResults = [select Status, count(Id) from CampaignMember where CampaignId = :camp.id group by status];
// Loop through the query and add chart items
for (AggregateResult ar : groupedResults) {
items.add(new ChartDataItem( String.valueOf(ar.get('Status')), String.valueOf(ar.get('expr0'))));
}
Now we are doing everything we need in 1 query. Even if we had 1000 different member statuses, it wouldn’t matter. We should never hit a governor limit with this code and performance has also been improved. Some important things to note in making this change and in understanding aggregate functions:
- Your controller class should be on API version 18.0 or higher. 18.0 is the Spring ’10 release and that’s when these functions were introduced.
- The results of a query with aggregate functions should result in a AggregateResult[] collection.
- The get() method is used to retrieve data from an AggregateResult object (used inside the loop when looking at a single result)
- To get the value from a field your are grouping by (i.e. doesn’t have a function for it), use .get(‘fieldName’). In the above example, I use this to get the Status value I am grouping by.
- To get the value from a field that has an aggregate function, use .get(‘expr#’), where # is the index number for that field. A query can have multiple functions in it so you need to specify which function you are grabbing and you do so by its index number. For you non-programmers out there, remember that counting starts at 0. In the above example, I use this to get the count(id) value. Since I only have 1 aggregate function in my query, i get it using .get(‘expr0’) where 0 is the index number for my function result.
- The get() method returns an Object type so you will need to use the appropriate valueOf() method to put it into the data type you need.
NOTE: As of writing this post, the IDE was not yet upgraded to 18.0 so I had to do this work inside the browser and I used the new-ish Deploy functionality from inside Salesforce to migrate the change from Sandbox to Production.